Introduction
This section will show a simple example of using the HttpListeningHandler
to post to PeopleSoft
using a synchronous service operation. This is the request/reply paradigm where
code is run in response to in inbound message.
In this article:
- We are posting
to an Synchronous Service operation in PeopleSoft
- The PeopleCode that is executed will examine the XML sent and do something different depending on the content. The response will also be different based on the input.
Detail view:-
- Create a New
PeopleSoft Message Object: Navigation: PeopleTools >
Integration Broker > Integration Setup > Messages
- Create a new
Service: Navigation: PeopleTools > Integration Broker
> Integration Setup > Service
- Create the
Handler PeopleCode
- Setup new
Synchronous Service Operation - Version, Routing and Service Operation Security
- Assign Service
Handler Class into Service Operation
- Test
Create a New PeopleSoft Message Object
Now we need a PeopleSoft message object that will represent the XML that the client program will post.
Message Attribute | Value |
---|---|
Name | CHG_GENERIC |
Version | V1 |
Type | Nonrowset-based |
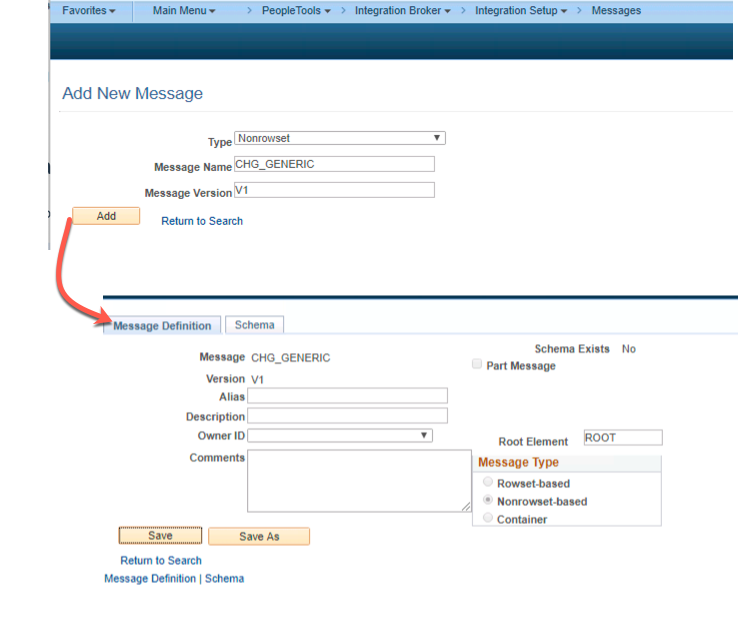
Create a new Service
In this example, we create a new service. You can easily re-use another service if you wish.
Service Attribute | Value |
---|---|
Name | CHG_TEST_SYNC |
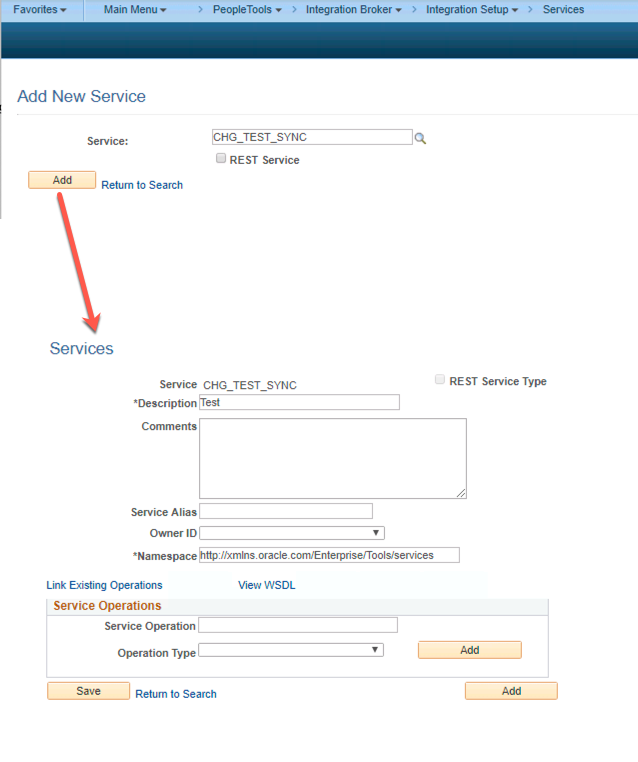
Create the Handler PeopleCode
Now we need to create some PeopleCode that will run when a new message is posted to the integration broker for this Service Operation.
- Create an Application Package and Class with the following Package Class Name:
CHG_SYNC_TEST:syncTest
- Paste in the following code into the Class:
import PS_PT:Integration:IRequestHandler;
class syncTest implements PS_PT:Integration:IRequestHandler
method onRequest(&MSG As Message) Returns Message;
end-class;
method onRequest
/+ &MSG as Message +/
/+ Returns Message +/
/+ Extends/implements PS_PT:Integration:IRequestHandler.OnRequest +/
Local XmlDoc &xmlDocFromPython;
Local XmlNode &requestRootNode;
&xmlDocFromPython = &MSG.GetXmlDoc();
&requestRootNode = &xmlDocFromPython.DocumentElement;
/* Setup response xml body */
Local Message &response;
&response = CreateMessage(Operation.CHG_SYNC_TEST, %IntBroker_Response);
Local XmlDoc &xmlout;
Local XmlNode &childNode;
&xmlout = CreateXmlDoc("<?xml version='1.0'?><response/>");
Evaluate Lower(&requestRootNode.NodeName)
When = "helloworld"
&childNode = &xmlout.DocumentElement.AddElement("helloworld").AddText("Hello client");
Break;
When = "activeusercount"
Local integer &ucount;
SQLExec("SELECT COUNT(*) FROM PSOPRDEFN WHERE ACCTLOCK = 0 ", &ucount);
&childNode = &xmlout.DocumentElement.AddElement("activeusercount").AddText(String(&ucount));
Break;
When-Other
&childNode = &xmlout.DocumentElement.AddElement("error").AddText("I do not understand. Please try again. You submitted: " | &requestRootNode.NodeName);
Break;
End-Evaluate;
&response.SetXmlDoc(&xmlout);
Return &response;
end-method;
- A synchronous request must implement the
OnRequest
method. - You will see that the code examines the Root Node of the XML and will run a different branch of code depending on the input.
- “helloworld” - If the root node is “helloworld” we will just echo back a “Hello client” message.
- “activeusercount” - If the root node is “activeusercount”, then we will do a count of all unlocked users in the system and return that in a response
- If any other root node is passed in we return an error message that the input was not understood and we echo back the root node name in the message.
This will probably make a little more sense later in the article when we look at the request and response from the Python client.
Setup new Synchronous Service Operation
Now we need to setup the actual Service Operation. There are several steps here.
PeopleTools > Integration Broker > Service Operation Monitor > Administration > Domain StatusService Operation Attribute Value Name CHG_SYNC_TEST Type Synchronous Version V1 Active Checked Message Version CHG_TEST.V1 Queue Name IB_EXAMPLES (or create a new queue )
- Click on the “Service Operation Security” link
- Input a permission list that you have on your user profile.
- Additionally, assign permission lists grants to a permission list that is on your “default user id” from your node definition.
Now we need to hook the CHG_I_TESTER:syncTester
application class to execute when a service operation is posted. We do this on the Handler tab of the Service Operation.
Service Operation Handler Attribute Value Handler Name Test (This value will be over written by peoplecode) Handler Type OnRequest Implementation Application Class Description Tester Package Name CHG_I_TESTER Path : Class ID syncTest Method OnRequest
Now we need to setup the routing to make this node able to send Service Operations.
Service Operation Routing Attribute Value routing name CHG_IN_SYNC_TEST sender node CHG_TEST_NODE Receiver Node PSFT_CS (or whatever your default local node is ) External Alias CHG_SYNC_TEST.V1 Active Checked
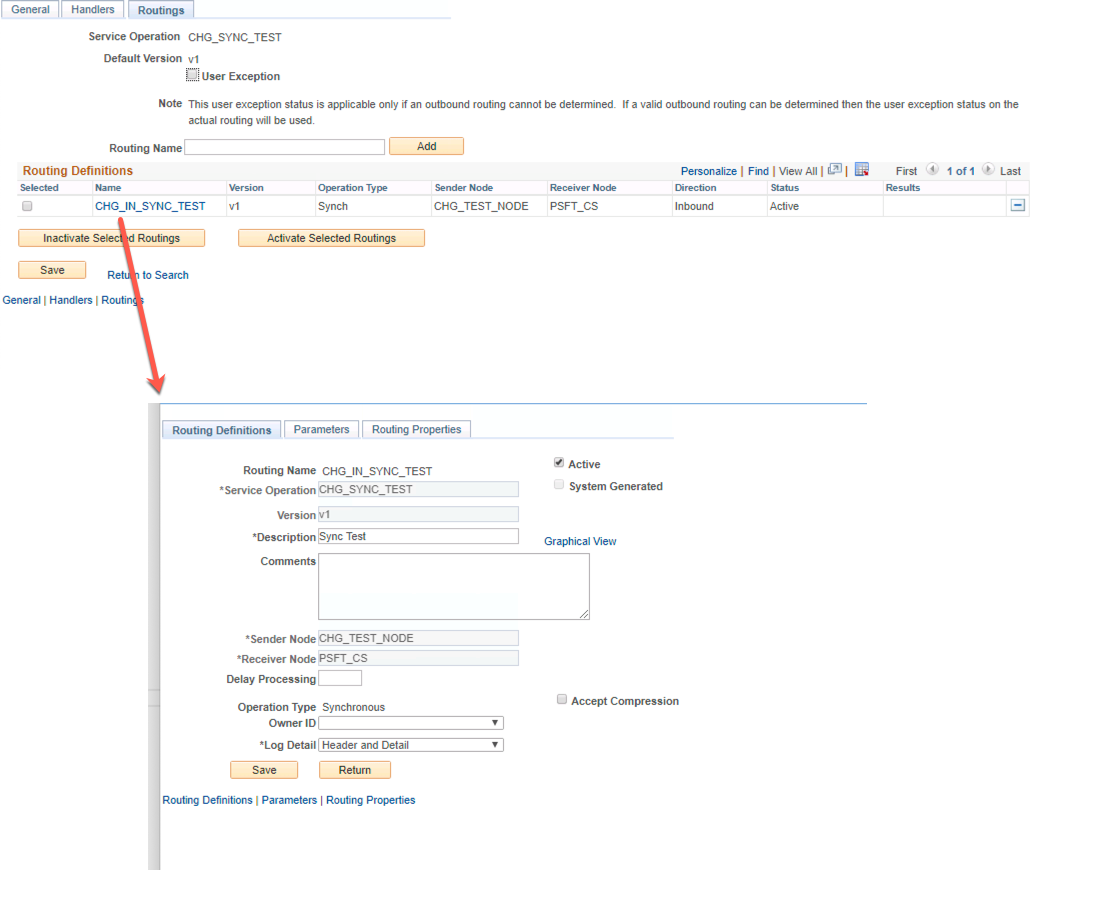
No comments:
Post a Comment